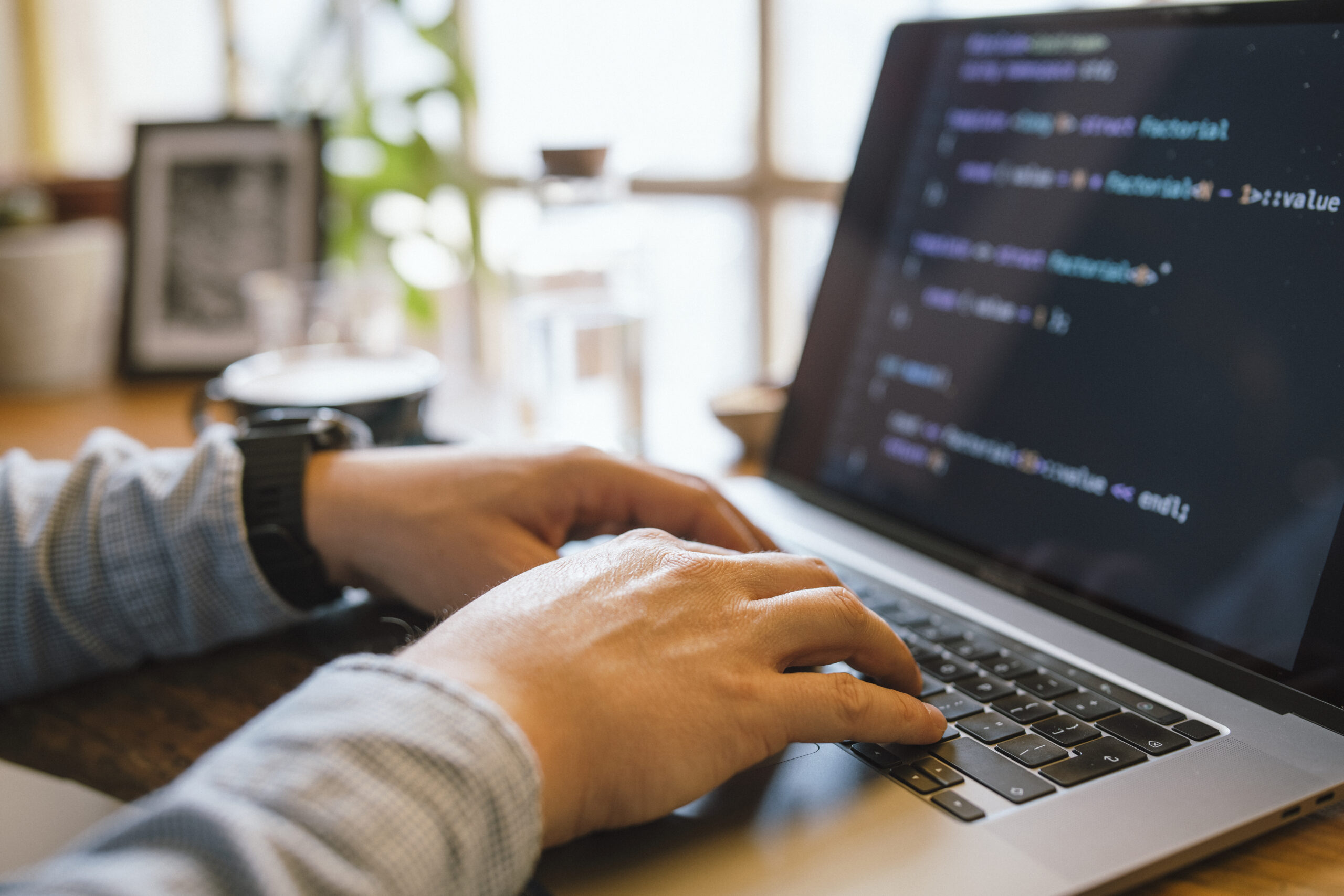
Debugging is Probably the most important — still normally overlooked — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about understanding how and why things go Incorrect, and Studying to Feel methodically to solve difficulties proficiently. No matter if you are a starter or a seasoned developer, sharpening your debugging abilities can conserve hrs of irritation and significantly increase your efficiency. Here i will discuss several strategies to assist developers level up their debugging sport by me, Gustavo Woltmann.
Master Your Tools
One of the quickest ways builders can elevate their debugging techniques is by mastering the instruments they use every single day. Although composing code is one particular Section of advancement, understanding how to connect with it efficiently throughout execution is Similarly critical. Modern day development environments appear Outfitted with powerful debugging abilities — but numerous builders only scratch the surface area of what these tools can perform.
Consider, for example, an Built-in Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to established breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code on the fly. When applied properly, they Permit you to observe particularly how your code behaves throughout execution, and that is priceless for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for front-stop builders. They permit you to inspect the DOM, watch network requests, look at serious-time functionality metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can transform aggravating UI issues into manageable tasks.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging procedures and memory management. Mastering these equipment may have a steeper Finding out curve but pays off when debugging efficiency issues, memory leaks, or segmentation faults.
Over and above your IDE or debugger, turn into snug with version Regulate units like Git to know code historical past, come across the precise moment bugs have been launched, and isolate problematic improvements.
Finally, mastering your tools indicates going past default options and shortcuts — it’s about establishing an personal familiarity with your progress natural environment to make sure that when issues arise, you’re not lost in the dark. The better you know your tools, the greater time you could expend resolving the particular challenge in lieu of fumbling by the procedure.
Reproduce the condition
One of the more important — and sometimes neglected — methods in successful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers want to create a dependable natural environment or circumstance exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of probability, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is accumulating as much context as feasible. Check with inquiries like: What actions triggered The difficulty? Which setting was it in — advancement, staging, or output? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it will become to isolate the exact disorders beneath which the bug occurs.
When you finally’ve collected plenty of info, seek to recreate the trouble in your neighborhood surroundings. This may imply inputting a similar info, simulating similar consumer interactions, or mimicking system states. If The problem seems intermittently, think about composing automatic exams that replicate the sting conditions or state transitions included. These checks not just enable expose the problem but in addition reduce regressions in the future.
Often, The difficulty may be setting-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Applying equipment like Digital devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It needs persistence, observation, plus a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. Using a reproducible circumstance, You should utilize your debugging instruments additional successfully, check prospective fixes securely, and talk a lot more Obviously along with your group or end users. It turns an abstract grievance into a concrete challenge — Which’s where by builders prosper.
Go through and Realize the Error Messages
Error messages are often the most beneficial clues a developer has when a little something goes Completely wrong. In lieu of observing them as annoying interruptions, developers should learn to take care of error messages as direct communications from the procedure. They generally let you know precisely what happened, wherever it took place, and occasionally even why it happened — if you know the way to interpret them.
Start out by reading through the message diligently and in complete. Lots of developers, especially when underneath time strain, glance at the 1st line and right away start building assumptions. But deeper during the error stack or logs may lie the genuine root trigger. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can guidebook your investigation and issue you towards the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically abide by predictable patterns, and Mastering to acknowledge these can dramatically hasten your debugging process.
Some problems are imprecise or generic, As well as in These circumstances, it’s very important to examine the context through which the mistake happened. Examine similar log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger concerns and supply hints about probable bugs.
Ultimately, error messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more impressive tools in a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s happening under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with knowing what to log and at what amount. Popular logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts all through progress, Data for basic occasions (like successful start-ups), Alert for likely concerns that don’t break the applying, ERROR for real troubles, and FATAL in the event the process can’t keep on.
Steer clear of flooding your logs with too much or irrelevant facts. Excessive logging can obscure essential messages and slow down your process. click here Concentrate on vital functions, state variations, input/output values, and critical final decision points in the code.
Format your log messages Evidently and persistently. Consist of context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all devoid of halting the program. They’re In particular valuable in generation environments where stepping by way of code isn’t possible.
Moreover, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about equilibrium and clarity. Using a very well-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly establish and take care of bugs, developers should technique the method similar to a detective resolving a secret. This mindset assists break down sophisticated troubles into workable sections and follow clues logically to uncover the root lead to.
Start out by accumulating evidence. Look at the signs of the challenge: mistake messages, incorrect output, or effectiveness concerns. The same as a detective surveys a criminal offense scene, accumulate just as much suitable facts as you could without the need of leaping to conclusions. Use logs, exam conditions, and person experiences to piece jointly a transparent photo of what’s occurring.
Upcoming, sort hypotheses. Question by yourself: What may very well be resulting in this habits? Have any adjustments not too long ago been created towards the codebase? Has this problem occurred right before underneath very similar instances? The intention will be to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the issue in a managed surroundings. If you suspect a selected operate or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out close notice to tiny details. Bugs generally conceal during the minimum envisioned areas—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be complete and affected person, resisting the urge to patch The difficulty without having absolutely comprehension it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging method can help you save time for long term troubles and help Other individuals fully grasp your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in advanced systems.
Compose Exams
Writing exams is one of the best solutions to help your debugging abilities and All round growth performance. Checks don't just help catch bugs early but in addition serve as a safety Internet that provides you self esteem when building variations to your codebase. A well-tested application is easier to debug because it allows you to pinpoint precisely exactly where and when an issue occurs.
Start with unit checks, which focus on individual capabilities or modules. These compact, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as predicted. Each time a check fails, you instantly know exactly where to look, significantly lessening some time expended debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming preset.
Upcoming, combine integration exams and end-to-stop assessments into your workflow. These aid make certain that numerous aspects of your software perform together effortlessly. They’re specially valuable for catching bugs that take place in complex devices with several components or expert services interacting. If one thing breaks, your checks can inform you which part of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to Imagine critically regarding your code. To check a attribute properly, you require to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug can be a strong starting point. Once the examination fails continuously, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that the exact same bug doesn’t return Down the road.
In brief, crafting tests turns debugging from a annoying guessing activity into a structured and predictable procedure—supporting you capture extra bugs, quicker and even more reliably.
Acquire Breaks
When debugging a tough difficulty, it’s simple to become immersed in the trouble—watching your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your head, lower irritation, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too very long, cognitive tiredness sets in. You could possibly start off overlooking clear glitches or misreading code you wrote just several hours previously. In this particular condition, your brain becomes less economical at trouble-resolving. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your emphasis. A lot of developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the background.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Electricity as well as a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that point to move all over, stretch, or do anything unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, having breaks isn't a sign of weak spot—it’s a smart method. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to develop like a developer. No matter if it’s a syntax mistake, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing worthwhile when you take the time to reflect and evaluate what went Improper.
Start off by inquiring on your own a handful of key concerns once the bug is resolved: What induced it? Why did it go unnoticed? Could it are already caught before with superior techniques like device tests, code opinions, or logging? The responses generally expose blind places with your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a great behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or widespread problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends might be Specifically potent. Whether it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the similar concern boosts team performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a few of the most effective developers are usually not the ones who produce ideal code, but people that constantly master from their blunders.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.